Secure Fields
Secure Fields offer you maximum design freedom with minimal compliance effort. Secure Fields are secure iFrames which you can use to capture card information from your customers while also ensuring sensitive card data never comes into contact with your systems. Two iFrames will have to be injected into your page: One for the card number and another for the card security code. Your systems will never touch any sensitive information and you will be able to keep almost all card payment interactions on your page. While this integration requires more effort on your side compared to our Redirect or Lightbox integration, you can offer a seamless payment experience for card payments by fully integrating the payment forms in your checkout. The browser versions that support our Secure Fields are listed below.
![]() | ![]() | ![]() | ![]() |
Only card payments are supported
Our Secure Fields integration support card payments only. Please check the payment method overview to see which cards are currently supported.
Process overview
To start a transaction via our Secure Fields integration, you will need to call our secureFieldsInit endpoint ¹ to receive a transactionId
². For any new operation, you should retrieve a new transactionId. A transactionId is valid for 30 minutes. The transactionId can now be sent to your frontend and used to start the Secure Fields Javascript library ³. Once your customer completes the required information in your UI, you can submit the form's content ⁴. After submitting the form we will inform you if 3D Secure is required or not. If 3D Secure is required to complete the payment, you will have to redirect the customer to a specific Datatrans URL which will finally redirect to the cardholder's bank (ACS) ⁵. Upon completion of the 3D Secure authentication, we will automatically redirect the customer to the returnUrl
specified in the secureFieldsInit request. To fully authorize the transaction, call the split-authorize endpoint ⁶ from your backend.
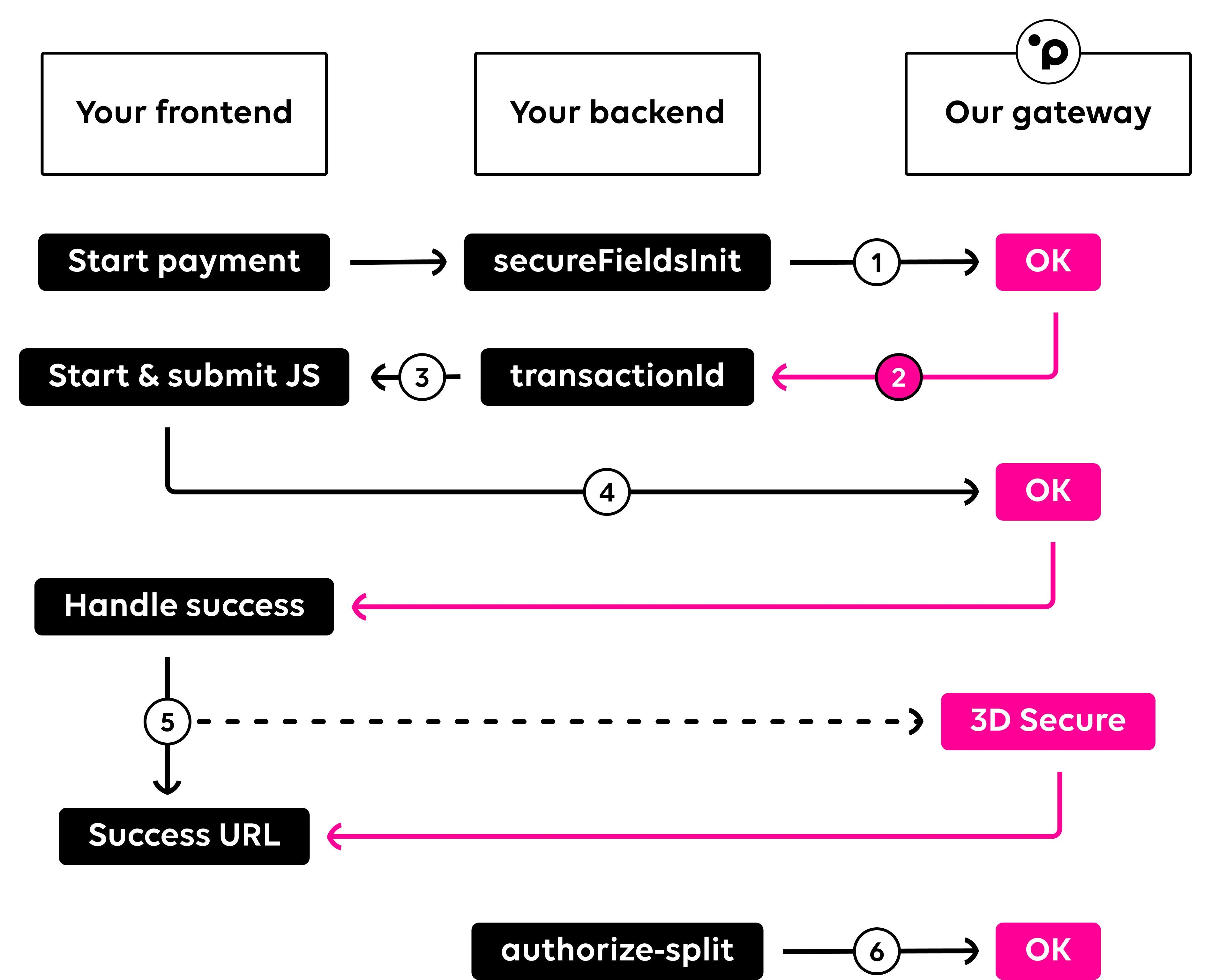
Secure Fields integration
To get started include our Secure Fields script on your web page.
<script src="https://pay.sandbox.datatrans.com/upp/payment/js/secure-fields-2.0.0.min.js"></script>
Next, you'll want to insert the card number and card security code iFrames at your desired location. Create empty DOM elements and assign a unique CSS ID to each of them. In the example, the unique IDs are card-number-placeholder
and cvv-placeholder
.
<form>
<div>
<div>
<label for="cardNumberPlaceholder">Card Number</label>
<!-- card number container -->
<div id="cardNumberPlaceholder" style="width: 250px;"></div>
</div>
<div>
<label for="cvvPlaceholder">Cvv</label>
<!-- cvv container -->
<div id="cvvPlaceholder" style="width: 90px;"></div>
</div>
<button type="button" id="go">Pay!</button>
</div>
</form>
Initializing transactions
To create a payment with Secure Fields, you will need to initialize a transactionId
via a server-to-server call first. Call our secureFieldsInit endpoint with your desired parameters and we'll return a transaction identifier ready to be used with our Secure Fields. The parameter returnUrl
specifies where the browser should be redirected to after a 3D Secure authentication. Please note that a transactionId
is only valid for 30 minutes. If you wish to do a dedicated registration, you must omit the parameter amount
. Please read our section saving payment information for more details.
curl 'https://api.sandbox.datatrans.com/v1/transactions/secureFields' \
--header 'Authorization: Basic {basicAuth}' \
--header 'Content-Type: application/json' \
--data-raw '{
"amount": 100,
"currency": "CHF",
"returnUrl": "{returnUrl}"
}'
Initializing our Javascript library
Now that you have a transactionId
, you can initialize the Secure Fields JavaScript library and specify which DOM element containers should be used to inject the iframes.
var secureFields = new SecureFields();
secureFields.init(
"transactionId", {
cardNumber: "cardNumberPlaceholder",
cvv: "cvvPlaceholder",
});
Submitting your form
Use the submit function to submit the card information. Don't forget to also submit the expiry month and the expiry year of the card. These two items are not subject to PCI compliance, so you will have to collect them without injecting our iFrames. Secure Fields collect expiry data provided by the credit card autofill feature of modern browsers and password managers. See Secure Fields Events - Browser autofill.
secureFields.submit({
expm: 6,
expy: 25
});
Success & 3D Secure
Within the event success
you can handle the data received after the secureFields.submit()
call. If 3D authentication is required for a specific transaction, the parameter redirect
inside the object data
will indicate the URL that the customer needs to be redirected to. This URL is provided by the cardholder's bank (ACS). Upon completion of the 3D Secure authentication, we will automatically redirect the customer to the returnUrl
specified in the secureFieldsInit request. The returnUrl
will also contain a parameter called status_3d
stating the result of the 3D Secure authentication process. The possible authentication response values are outlined in the 3D Secure section in our docs.
Below is an example of the success event data. Refer to the section Secure Fields Events to see all possible events.
{
"event":"success",
"data": {
"transactionId":"{transactionId}",
"cardInfo": {
"brand":"MASTERCARD",
"type":"credit",
"usage":"consumer",
"country":"CH",
"issuer":"DATATRANS"
},
"redirect":"https://pay.sandbox.datatrans.com/upp/v1/3D2/{transactionId}"
}
}
Finalizing the authorization
To finalize any transaction with our Secure Fields, call our authorize-split endpoint. Here you can specify if the transaction should be settled automatically or if you wish to proceed with a deferred settlement.
curl 'https://api.sandbox.datatrans.com/v1/transactions/{transactionId}/authorize' \
--header 'Authorization: Basic {basicAuth}' \
--header 'Content-Type: application/json' \
--data-raw '{
"refno": "Test-1234",
"amount": 100,
"autoSettle": true
}'
Mandatory 3DS Data
We require various data fields for 3DS authentication processing. Your init requests must include valid consumer data, including the cardholder's name, email, and phone number. For more information, please refer to the 3DS mandatory data overview.
{
...
"3D": {
"cardholder" : {
"cardholderName": "John Doe",
"email": "[email protected]",
"homePhone": {
"cc": "41",
"subscriber": "791234567"
}
}
}
}
You also have the option to pass the required 3D information during your secureFields.submit()
call, although we recommend passing this information via init whenever possible. Passing new information in this call will overwrite previously submitted information.
secureFields.submit({
expm: 6,
expy: 25,
"3D": {
"cardholder": {
"email": "[email protected]",
"cardholderName": "John Doe",
"homePhone": {
"cc": "41",
"subscriber": "123456789"
}
}
}
});
Currency Conversion
The Secure Fields integration supports dynamic currency conversion (DCC) and multi-currency pricing (MCP) of our Currency Conversion offering.
For DCC, you must pass a new configuration option dccEnabled
, listen to event on.('dccAvailable', ...)
and if returned, resubmit the secureFields with the dcc choice made by the consumer. You will remain responsible for listing the card and merchant currency options and submitting the form with the consumer's choice. Please refer to the dedicated page Dynamic currency conversion for more details.
For MCP, you must get the currency rates and pass an additional object mcp
in your secure fields init requests. Please refer to the dedicated page Multi-currency pricing for more details.
Showcase & more
Please refer to our Github sample for more detailed examples. Also check our the dedicated page Styling & Initialization Options to see what options you have to style and initialize the library. For the sake of simplicity, other important fields like amount, expiry, and cardholder name are omitted.
Updated 2 months ago