Mobile SDK
Accept payments on your iOS or Android apps: Our mobile SDKs support your entire payment and registration process and simplify the integration of any payment method in your mobile apps. Completely outsource your payment processes to us from inside your native apps. We also take care of redirecting your users to 3D Secure processes and enable smooth app switches to other payment applications including PayPal, Twint, and PostFinance. Check the release notes and the integration link of our iOS and Android SDK below.
App Clips (iOS), Instant Apps (Android), Elo, Hipercard, and Klarna!
The Mobile SDK for iOS and Android can now be linked to your projects with only third-party dependencies that are required in your project - that way the size of our SDK within your projects can be reduced. That will be useful for App Clips (iOS) and Instant Apps (Android)! Starting 2.2.0, the SDKs now also support tokenization flows for Elo & Hipercard, and Klarna for payments flows.
Send us a message anytime by using this link if you have questions about our new SDKs. If you want to read what was renewed, please click here.
Process overview
To start a transaction via our SDK, you will need to call our init endpoint ¹ to receive a mobileToken ². For any new operation you should retrieve a new mobileToken. A mobileToken is valid for 30 minutes. The mobileToken can now be sent to your mobile application and used to start the SDK ³. Once your customer completes the transaction ⁴ we will return the transaction information to your defined webhook and to the delegate ⁵ defined in your application.
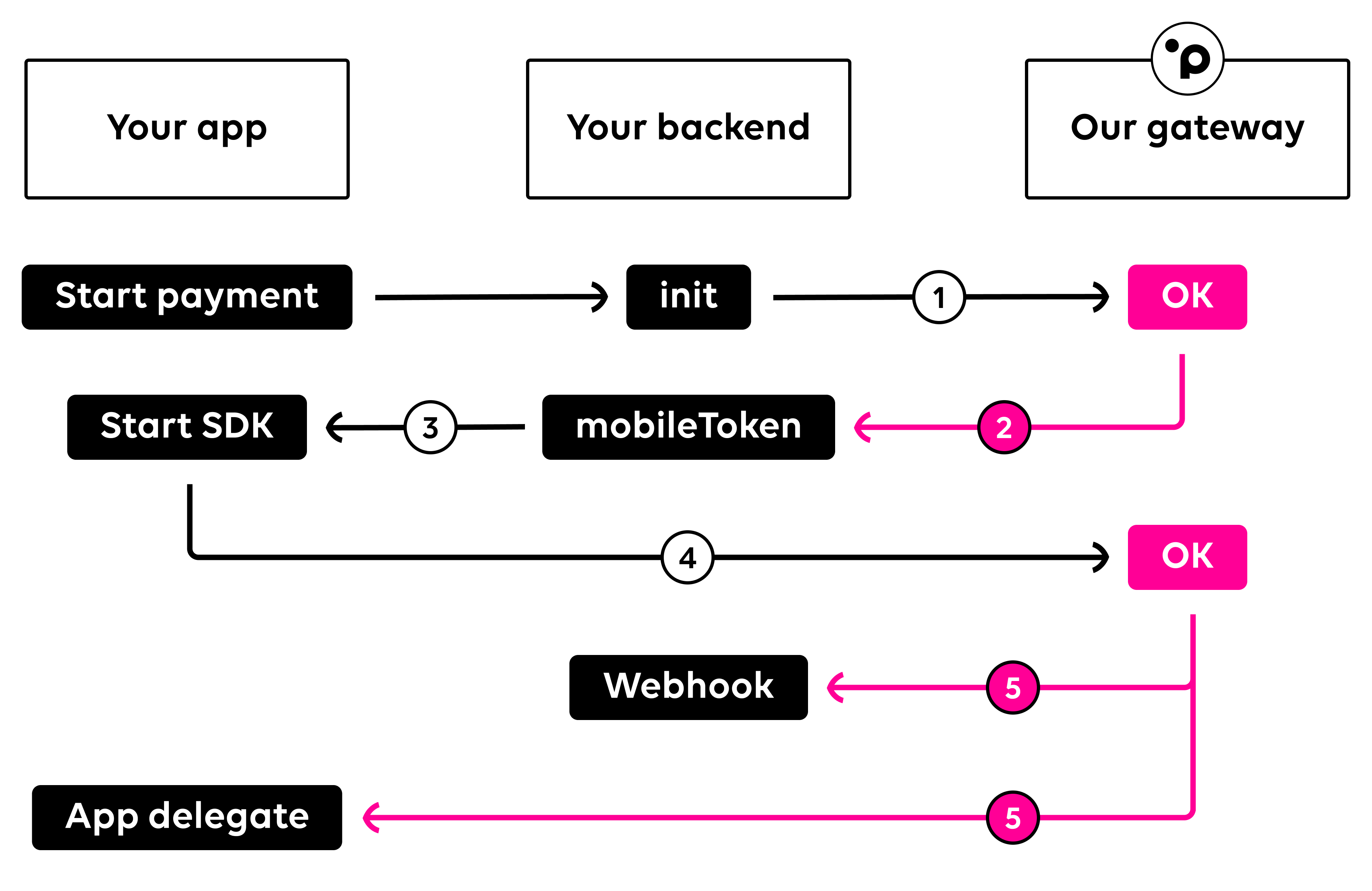
Initializing transactions
To create a payment with our Mobile SDKs, you will need to initialize a mobileToken
via a server-to-server call first. Call our init endpoint with your desired parameters and the option returnMobileToken
and we'll return a mobileToken
ready to be used with our SDKs. Please note that a mobileToken
is only valid for 30 minutes. If you wish to do a dedicated registration, you must omit the parameter amount
. Please read our section saving payment information for more details.
You need to specify at least one payment method during your init call to retrieve a mobileToken
. If you do the preselection of the payment method on your end, simply send the array paymentMethods
including only one payment method to directly show the final payment screen of that specific payment method. If you wish to display several payment methods on our Mobile SDK, you can simply extend the array with further payment methods. The order of the payment methods in your init call defines the order that is displayed in our SDKs.
If a payment method requires specific customer, shipping, or billing information, you may include it as well during your init payload. This is usually necessary for invoice payments.
curl 'https://api.sandbox.datatrans.com/v1/transactions' \
--header 'Authorization: Basic {basicAuth}' \
--header 'Content-Type: application/json' \
--data-raw '{
"currency": "CHF",
"refno": "Test-1234",
"amount": 100,
"paymentMethods": ["ECA","VIS","PAP","TWI"],
"option": {
"returnMobileToken": true
}
}'
Now that you have retrieved a mobileToken
, you can continue with the initialization of our iOS or Android SDK.
iOS integration
For iOS, you can add the SDK to your project by adding a new Swift package dependency in Xcode and pointing to the github repository github.com/datatrans/ios-sdk
. By default, enable all packages presented in the package selection. If you are building an App Clip project, you may select only your required packages, which will depend on your payment methods and flows. Please contact our support if you are unsure about what packages you require. Besides adding the packages via a Swift package dependency, you can also add the SDK via Cocoapods with pod 'Datatrans'
.
Call the library with your mobile token to start a transaction. You can optionally specify further options and pass tokens for fast checkouts with our provided classes. Below is an example of the suggested minimum options to start a transaction with iOS (Swift, Objective-C). Please read our detailed class description for iOS to discover more initialization options.
let transaction = Transaction(mobileToken: String)
transaction.delegate = self // you must implement your TransactionDelegate here
transaction.options.testing = true
transaction.options.useCertificatePinning = true
transaction.start(presentingController: navigationController)
DTTransaction* transaction = [[DTTransaction alloc] initWithMobileToken:mobileToken ];
transaction.delegate = self; // you must implement your TransactionDelegate here
transaction.options.testing = YES;
transaction.options.useCertificatePinning = YES;
[transaction startWithPresentingController:self.navigationController];
Info.plist file
To integrate a card scanner into your iOS app, you must include a camera usage description in your Info.plist file. This explains to users why your app requires camera permissions. If you omit this description, your app might crash when using the card scanner feature.
Additionally, if you want to integrate alternative payment methods like Klarna, PostFinance, Swish, Twint, or Vipps, you'll need to configure your app to switch between your app and the respective payment app smoothly. For iOS, this involves adding the LSApplicationQueriesSchemes
array to your Info.plist and listing the necessary payment app schemes (see example below).
#CameraUsage
Key : Privacy - Camera Usage Description
Value : $(PRODUCT_NAME) requires camera access to scan cards.
#Klarna
Key : LSApplicationQueriesSchemes
Value : [ "klarna", "klarnaconsent" ]
#PostFinance
Key : LSApplicationQueriesSchemes
Value : [ "postfinance-epayment" ]
#Swish
Key : LSApplicationQueriesSchemes
Value : [ "swish" ]
#Twint
Key : LSApplicationQueriesSchemes
Value : [ "twint-extended", "twint-issuer1", ... , "twint-issuer39" ]
#Vipps
Key : LSApplicationQueriesSchemes
Value : [ "vipps", "vippsMT" ]
<key>NSCameraUsageDescription</key>
<string>$(PRODUCT_NAME) requires camera access to scan cards.</string>
<key>LSApplicationQueriesSchemes</key>
<array>
<string>klarna</string>
<string>klarnaconsent</string>
<string>postfinance-epayment</string>
<string>swish</string>
<string>vipps</string>
<string>vippsMT</string>
<string>twint-extended</string>
<string>twint-issuer1</string>
<string>twint-issuer2</string>
<string>twint-issuer3</string>
<string>twint-issuer4</string>
<string>twint-issuer5</string>
<string>twint-issuer6</string>
<string>twint-issuer7</string>
<string>twint-issuer8</string>
<string>twint-issuer9</string>
<string>twint-issuer10</string>
<string>twint-issuer11</string>
<string>twint-issuer12</string>
<string>twint-issuer13</string>
<string>twint-issuer14</string>
<string>twint-issuer15</string>
<string>twint-issuer16</string>
<string>twint-issuer17</string>
<string>twint-issuer18</string>
<string>twint-issuer19</string>
<string>twint-issuer20</string>
<string>twint-issuer21</string>
<string>twint-issuer22</string>
<string>twint-issuer23</string>
<string>twint-issuer24</string>
<string>twint-issuer25</string>
<string>twint-issuer26</string>
<string>twint-issuer27</string>
<string>twint-issuer28</string>
<string>twint-issuer29</string>
<string>twint-issuer30</string>
<string>twint-issuer31</string>
<string>twint-issuer32</string>
<string>twint-issuer33</string>
<string>twint-issuer34</string>
<string>twint-issuer35</string>
<string>twint-issuer36</string>
<string>twint-issuer37</string>
<string>twint-issuer38</string>
<string>twint-issuer39</string>
</array>
Twint requires you to define 40 schemes to support app switches to their many apps. If you have limited space due to other integrations (iOS has a limit of 50 schemes), use the SDK option twintMaxIssuerNumber
. Set this value to match your highest twint-issuerXY
scheme. For example, if Info.plist contains up to twint-issuer35
, set the SDK option twintMaxIssuerNumber
to 35
. Make sure the twint-extended
scheme is always included in your Info.plist file.
If possible, always make room for 40 Twint schemes!
Whenever possible, allocate the full 40 Twint schemes within
LSApplicationQueriesSchemes
. This ensures the best possible user experience, allowing for seamless app switching during payments.While the
twintMaxIssuerNumber
option can be used as a workaround if you face scheme limitations, it negatively impacts the user experience. Using this option prevents smooth automatic app switching during the Twint payment process.
Additional requirements for iOS
To enable Apple Pay in your iOS projects, you must create an Apple merchant identifier, upload a certificate to your Datatrans merchant settings and activate the Apple Pay capability in XCode. Please refer to the section Apple Pay via Mobile SDK to correctly enable Apple Pay for your XCode project.
To correctly process PayPal transactions in your iOS projects, you will need to include an additional component from PayPal called PPRiskMagnes
. To correctly process Klarna transactions, you will need to include the additional component from Klarna called KlarnaMobileSDK
. These are optional libraries if you link our SDK via Swift Package Manager. If you linked the SDK via CocoaPods, the component will automatically be added to your project.
If you are processing Twint, PostFinance Card, or PayPal please make sure to define your app callback scheme in the transaction options, as this is required to properly redirect users back to your app.
transaction.options.appCallbackScheme = "your_scheme"
transaction.options.appCallbackScheme = @"your_scheme";
Android integration
For Android projects, you can link the repo and dependencies via Gradle as demonstrated below.
repositories {
...
maven { url 'https://datatrans.jfrog.io/artifactory/mobile-sdk/' }
}
dependencies {
...
implementation 'ch.datatrans:android-sdk:3.1.0' // Check release notes for latest version
}
Call the library with your mobile token to start a transaction. You can optionally specify further options and pass tokens for fast checkouts with our provided classes. Below is an example of the suggested minimum options to start a transaction with Android (Kotlin, Java). Please read our detailed class description for Android to discover more initialization options.
val transaction = Transaction(mobileToken)
transaction.listener = this // this refers to Android's Activity
transaction.options.isTesting = true
transaction.options.useCertificatePinning = true
TransactionRegistry.startTransaction(this, transaction)
Transaction transaction = new Transaction(mobileToken);
transaction.setListener(this); // this refers to Android's Activity
transaction.getOptions().setTesting(true);
transaction.getOptions().setUseCertificatePinning(true);
TransactionRegistry.INSTANCE.startTransaction(this, transaction);
If you are building an Instant app, you may exclude specific packages that are not required in your project. This will depend on your payment methods and flows. Please reach out to our support if you are unsure about what packages you can exclude.
dependencies {
...
implementation ('ch.datatrans:android-sdk:3.1.0') { // Check release notes for latest version
exclude group: 'io.card', module: 'android-sdk'
}
}
The table below lists all the dependencies which can be excluded. Do not exclude a dependency if it is required by a payment method you included in your init request.
Feature | Group | Module |
---|---|---|
Credit Card Scanner | io.card | android-sdk |
Google Pay | com.google.android.gms | play-services-wallet |
PayPal | com.paypal.risk | android-magnessdk |
Samsung Pay | com.samsung.android.sdk | samsungpay |
Twint | ch.twint.payment | twint-sdk-android |
Additional requirements for Android
If you are integrating PayPal you must add various entries to your app manifest. You will run into technical issues if you do not define the requirements below.
For PayPal payments, an external web process is used. After this web process has finished, a callback to your app is issued. In order to receive this callback, you need to define the Datatrans relay activity with an intent filter in your AndroidManifest.xml
for a defined scheme. Keep in mind that the URI scheme must be unique to the shopping app and the activity. Do not use actual protocols or file types such as http
, mailto
, pdf
etc., or generic names such as ticket
. An example would be the package name extended by an identifier dtsdk
.
<activity
android:name="ch.datatrans.payment.ExternalProcessRelayActivity"
android:launchMode="singleTask"
android:enabled="false"
android:theme="@android:style/Theme.Translucent.NoTitleBar">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="your.package.name.dtsdk" />
</intent-filter>
</activity>
If you are processing Twint, PostFinance Card, or PayPal please make sure to define your app callback scheme in the transaction options, as this is required to properly redirect users back to your app.
transaction.options.appCallbackScheme = "your_scheme"
transaction.getOptions().setAppCallbackScheme("your_scheme");
To offer Klarna as a payment method, you must manually add the repository and dependency to your build gradle. This is currently the only payment method that requires you to do this manually.
repositories {
...
maven { url 'https://x.klarnacdn.net/mobile-sdk/' }
}
dependencies {
...
implementation 'com.klarna.mobile:sdk:2.1.8'
}
Auto settlements
Settle your transactions automatically or in two steps with the parameter autoSettle
during your init calls. If you do not specify anything in your init request, your transactions will not be settled automatically. Please refer to the section settling a transaction for more information on deferred settlements and the related server to server request.
"autoSettle": true
Saving payment information
Save card or payment method details after your customer has finalized their payment or registration. Not all payment methods support a registration with a payment. Please refer to our tokenization overview to see which payment methods require a dedicated registration. Please also read our section saving payment information for more details. Payment methods that do not support tokenization will be automatically omitted.
The payment method information will be returned by the SDK, in our webhook, and can be additionally retrieved by checking the status of a transaction.
"amount": 1000, // Omit the parameter amount for dedicated registrations
"option": {
"createAlias": true // Required to save a card (not needed for dedicated registrations)
}
If needed, you can convert the returned SavedPaymentMethod
class to a JSON object by using the function toJson
.
Fast checkouts
Fast checkouts or one-click checkouts can also be performed with our SDKs. For card payments, include the object card
with alias
, expiryMonth
, and expiryYear
in your init payload and we will continue with the authorization automatically once the SDK was initialized. This is useful for payments where you want your customers to complete a transaction quickly while also supporting 3D Secure. For other payment methods (eg. Twint, PayPal, or PostFinance Card) include the object of the payment method (eg. TWI
) and the corresponding alias
.
Only one payment method should be included in the array paymentMethods
. You may also avoid calling the SDK with aliases for this to work properly.
"card": {
"alias": "{cardAlias}",
"expiryMonth": "12",
"expiryYear": "25"
},
"paymentMethods": ["VIS"]
"TWI": {
"alias": "{{twintAlias}}"
},
"paymentMethods": ["TWI"]
You will achieve the same logic, if you submit only one alias within the SDK in the SavedPaymentMethod
class and its sub-classes. The implementation of the saved payment methods selection is explained below.
Saved payment method selection
Unlike our Redirect and Lightbox integration, you can call our SDK with previously saved payment methods (alias). You can even include multiple aliases per card brand (eg. two Visa aliases). This is useful if you want the user to finalize their payment with one click with a previously registered card. To initialize the library with an alias, you may refer to the class SavedPaymentMethod
and its sub-classes (eg. SavedCard
) for payment methods requiring more than just the alias to create a payment.
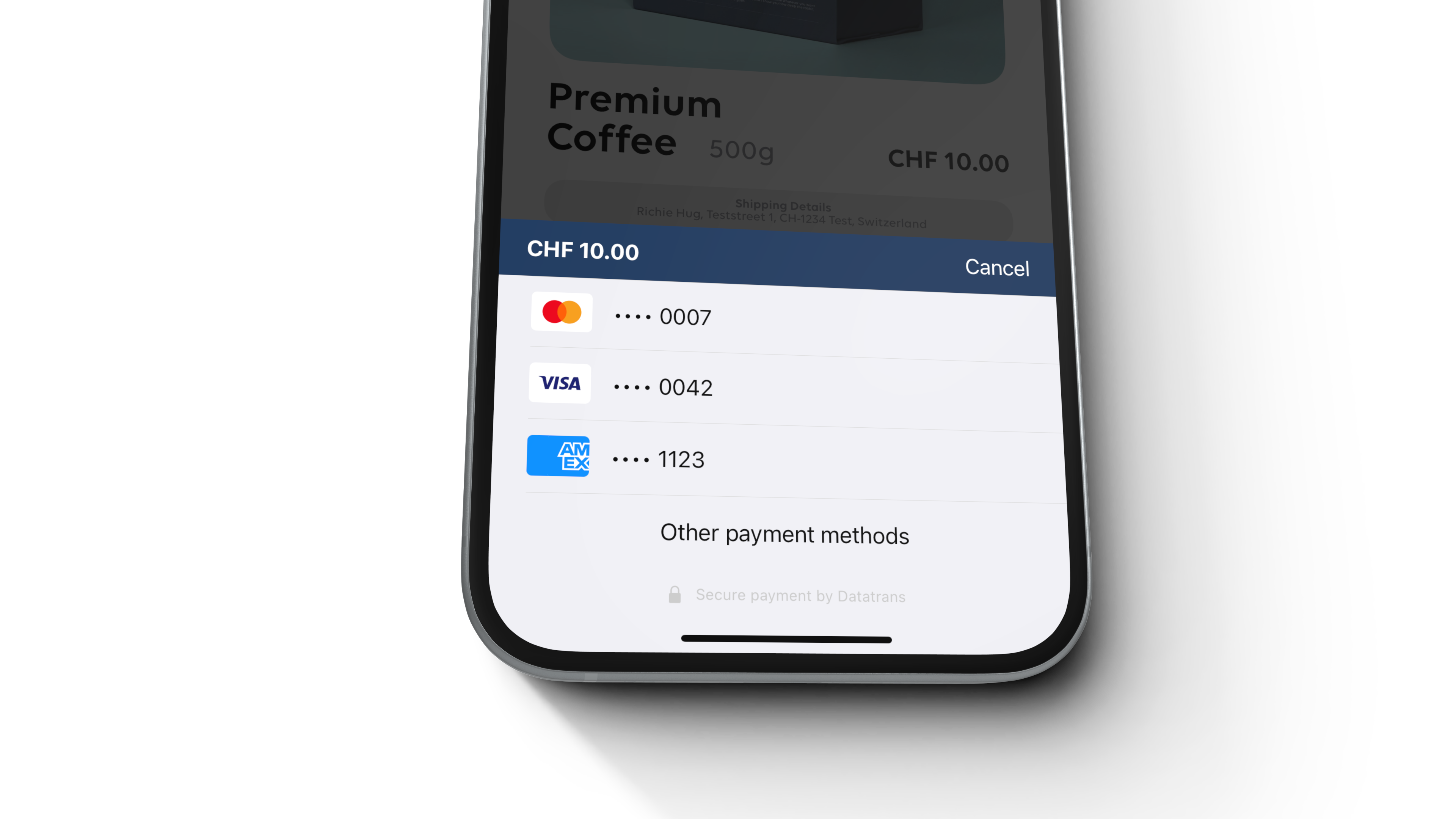
After the transaction
After the transaction has been completed, you can refer to the class TransactionSuccess
that will return the details of the transaction, including the payment method, the transaction unique identifier, and the token if one was created. If the transaction failed, you will have to refer to the class TransactionError
for further details instead. You may also implement TransactionDelegate
(iOS) and TransactionListener
(Android) to be notified when a transaction is successfully finalized, encounters an error, or is canceled by the user. We will also return the transaction information to your defined webhook.
Mandatory 3DS Data
We require various data fields for 3DS authentication processing. Your init requests must include valid consumer data, including the cardholder's name, email, and phone number. For more information, please refer to the 3DS mandatory data overview.
{
...
"card": {
"3D": {
"cardholder" : {
"cardholderName": "John Doe",
"email": "[email protected]",
"homePhone": {
"cc": "41",
"subscriber": "791234567"
}
}
}
}
}
Currency Conversion
The Mobile SDK integration supports dynamic currency conversion (DCC) and multi-currency processing (MCP) of our Currency Conversion offering. For DCC, you will not need to make any integration changes; it will be a simple change by us in your merchant configuration. Once DCC has been enabled for your merchantId, use savedCardDCCShowMode
in TransactionOptions
to enable DCC (default), disable DCC, or smartly enable DCC for transactions with saved cards (tokens). Smart DCC will trigger the DCC flow less frequently if the consumer declines the DCC choice in their previous choice. For MCP, you must get the currency rates and pass an additional object mcp
in your init requests. Please refer to the dedicated page Multi-currency processing for more details.
Language support
To change the language of our payment forms, you can pass the parameter language
during your init calls.
"language": "en"
Our iOS and Android Mobile SDKs currently support the following languages:
English en | Danish da | Dutch nl | Finish fi |
French fr | German de | Italian it | Norwegian no |
Portuguese pt | Spanish es | Swedish sv |
Please get in touch should you need an additional language to be added for your checkout or if you find a translation error.
Styling the theme
You can style various colors in our Mobile SDKs to match your corporate identity. Check the graphic below to see what color properties can be defined. On iOS, you can define your preferred colors within the class ThemeConfiguration
. On Android, set theme options in your project's color XML resource file, typically colors.xml. Use the color key names as defined in the table below. Don't forget to set colors for Android's light and dark theme (values
& values-night
).
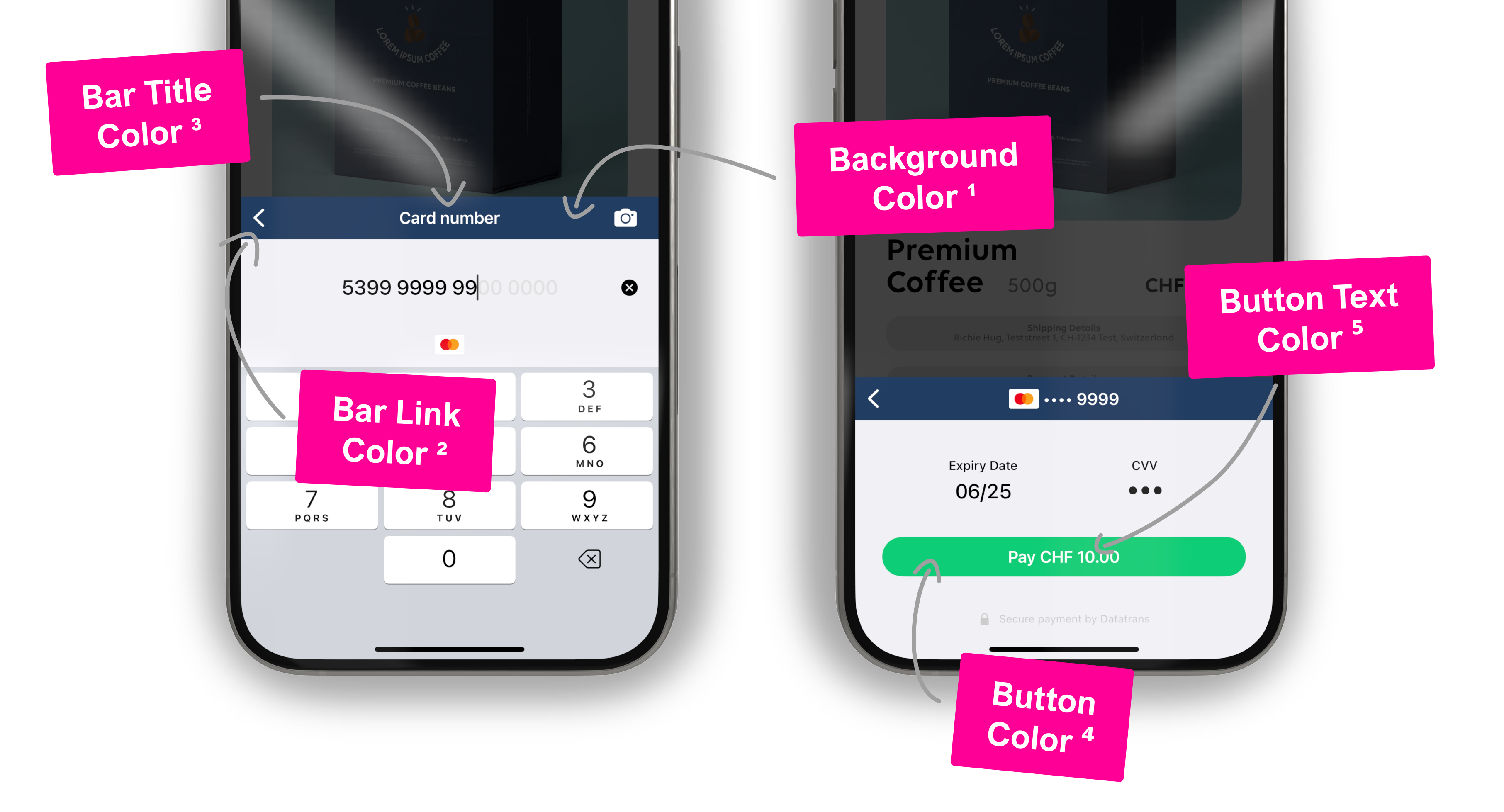
Property | Description | iOS | Android |
---|---|---|---|
Background Color¹ | Background color of the navigation bars. If this is not specified, the navigation bars will be transparent. | barBackgroundColor | dtpl_bar_background_color |
Bar Link Color² | Color of the buttons in the navigation bars. If this is not specified, the color will be the color set in Link Color. | barLinkColor | dtpl_bar_link_color |
Bar Title Color³ | Color of the title within the navigation bars. If this is not specified, the color will be the text color. The text color is either white or black and cannot be customized. | barTitleColor | dtpl_bar_title_color |
Button Color⁴ | Background color of large buttons, such as the Pay button. If this is not specified, the color will be the color set in Link Color. | buttonColor | dtpl_button_color |
Button Text Color⁵ | Text color of large buttons, such as the Pay button. If this is not specified, the color will be set to white. | buttonTextColor | dtpl_button_text_color |
Link Color⁶ | Color of text-only buttons or links and the text cursor. If this is not specified, the link color will be in a blue tone. | linkColor | dtpl_link_color |
Webhook
After any transaction that was processed via our Mobile SDK integration, we will call your webhook with all relevant transaction information. You can define your webhook URL within your merchant configuration. We include our mobileToken
in the HTTP header mobile-token
as well as a transactionId
in the payload to let you match Mobile SDK transactions. Any server to server request to modify an existing transaction has to be made with our transactionId
. Please refer to our page After The Payment for more information on actions you can make after a payment has been completed.
Within our SDK integration, your webhook will always be called synchronously after a transaction has been completed. Once your webhook has responded, the SDK will continue to the next state (success, error or cancel). We only try to call your webhook once. If it fails, no additional calls will be performed. Please let us know if we should call your webhook for registrations too as this is not active by default.
{
"transactionId" : "{transactionId}",
"merchantId": "1100012345",
"type" : "payment",
"status" : "authorized",
"currency" : "CHF",
"refno" : "Test-1234",
"paymentMethod" : "ECA",
"detail" : {
"authorize" : {
"amount" : 1000,
"acquirerAuthorizationCode" : "113009"
}
},
"card" : {
"alias" : "{cardAlias}",
"masked" : "520000xxxxxx0007",
"expiryMonth" : "12",
"expiryYear" : "29",
"info" : {
"brand" : "MCI CREDIT",
"type" : "credit",
"usage" : "consumer",
"country" : "MY",
"issuer" : "DATATRANS"
},
"3D" : {
"authenticationResponse" : "Y"
}
},
"history" : [ {
"action" : "init",
"amount" : 1000,
"source" : "api",
"date" : "20XX-09-14T09:25:27Z",
"success" : true,
"ip" : "85.0.141.184"
}, {
"action" : "authorize",
"amount" : 1000,
"source" : "redirect",
"date" : "20XX-09-14T09:25:26Z",
"success" : true,
"ip" : "85.0.141.184"
} ]
}
Updated 16 days ago